Objects and classes are the building blocks of Java programming language. Numerous FAQs in Java interviews are based on your knowledge of Java classes and objects; on topics such as access modifiers, non-access modifiers, classes, class members, extension of classes, abstract classes, interfaces, constructors, overriding and overloading of methods etc.
A thorough understanding of java classes and objects is absolutely necessary for being successful in your your Java interview; irrespective of the level of position - beginner as well as for senior positions; and for any role - programmer, designer, architect or manager.
The Object class is the root of the Java class hierarchy. Due to its significance, interview questions are frequently asked on the Object class and its methods. Some of below questions address this topic in detail.
Important keywords are provided at the end of below questions for your review.
What is Object class in Java programming language?
FAQKey Concept* Similar Java Interview Question Recently Asked @ Accenture, Citi, Expeditors, GlobalLogic, Google, Morgan Stanley, Oracle, Software AG, Vanguard,
Object class defined in java.lang package is the superclass of all other classes defined in Java programming language. Every class extends from the Object class either directly or indirectly. All classes inherit the instance methods defined in the Object class.
What are the non-static methods defined in the Object class that are inherited by all classes?
FAQKey Concept* Similar Java Interview Question Recently Asked @ Amadeus, Amdocs, Campus Labs, Deloitte, Ducat, Expeditors, FieldEZ, Goldman Sachs, Google, PWC, Rakuten, VirtUSA, Yodlee,
Object class defines eight non-static methods that are inherited by all other classes. A Java class can override any of these eight methods.
- clone() - If a class implements cloneable interface, then calling clone() method on its object returns a copy of the object. If a class does not implement the cloneable interface, and clone() method is called on its object, then the method throws a CloneNotSupportedException exception.
- toString() - You can get a string representation of any Java object by calling its toString() method. The toString() method is defined in the Object class and is inherited by all Java classes. The toString() method is usually overridden so that it returns a meaningful string representation of the object.
- equals() - You can check if an object equals another object by calling its equals() method and passing another object as a parameter to compare for equality.
The equals() method defined in the Object class uses the identity operator (==) to determine if two objects are equal. Hence this method returns correct result for primitive data types; but returns incorrect result for objects since the identity operator checks if the object references are equal instead of checking the logical equality of objects.
Hence equals() method is usually overridden to compare the logical equality of objects rather than their references.
- hashcode() - You get the hash code of an object by calling its hashcode() method. An objects hash code determines its memory address.
As a rule, if two objects are equal then their hash codes must also be equal. If you override equals() method, then you also have to override the hashcode() method to ensure that objects that are equal will have the same hash codes.
- finalize() - The Java garbage collector calls the finalize() method of an object just before the object is garbage collected. But you cannot control when, or if, an object is garbage collected. Hence, you should not rely on this method to perform critical tasks.
- getClass() - Calling getClass() method on an object returns a Class object which is a runtime class of this object. The Class class defines a number of methods which helps to find the metadata of a class - such as the class name, methods defined in the class, check if the class is an interface, check if the class is an interface etc.
- wait() - wait() is one of the three methods defined in Object class that facilitates thread to thread communication in multi-threaded Java programs. Calling wait() on an object of type Thread stops the execution of current thread until the processing of the other thread is complete.
- notify() - notify() is the second method defind in Java object class that facilitates thread to thread communication in multi-thread programming. notify() method will send an event notification or signal to a thread that is waiting in that object's waiting pool.
- notifyAll() - notifyAll() is the third method defind in Java object class that facilitates thread to thread communication in multi-thread programming. notifyAll() is similar to notify(), except that it sends notification to all threads that are waiting in that objects waiting pool
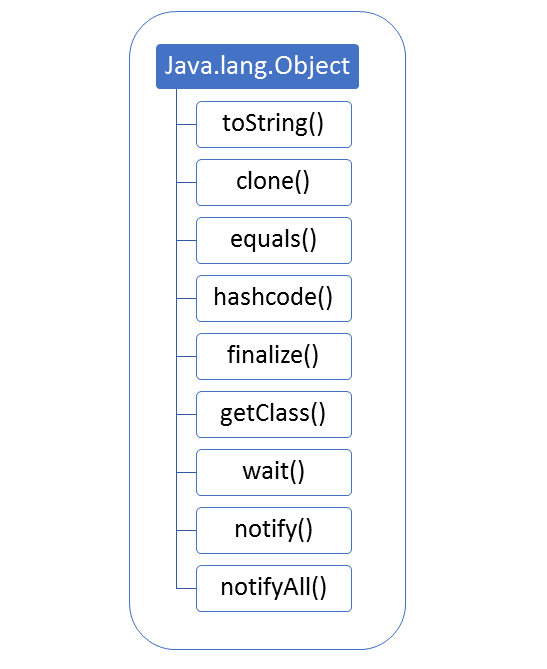
What is the difference between method Overloading and method Overriding?
FAQKey Concept* Similar Java Interview Question Recently Asked @ ACL Worldwide, Amadeus, American Express, Atos, CA Technologies, Campus Labs, Capital One, FGM Group, General Dynamics, Home Depot, IBM, Keysight Technologies, Northrop Grumman, Revaturte, TCS, Tech Mahindra, Techlogix, Thomson Reuters, TMC, Trustwave,
Methods having same name and return types but different arguments types can be declared within a class. These methods are called overloaded methods. This concept is known as method overloading
*** See complete answer and code snippet in the Java Interview Guide.
What are final classes and final methods Java programming language?
FAQKey Concept* Similar Java Interview Question Recently Asked @ Acceltree, Alcatel-Lucent, Antra, Apple, Aricent, Capgemini, Capttal One, C-Edge, ConnectWise, Faithlife, FDM, Financial Engines, GE, Goldman Sach, Group, Guidewire, HCL Technologies, IG, Incessant Technologies, Intuit, MathWorks, OpenEye, Oracle, Orbitz, Palo Alto Networks, Priceline, Rackspace, Realex Payments, Revature, Rolta, Sears, ServiceNow, SonicWall, TechFlow, Virtusa, VMWare, Wealthfront,
Final classes are Java classes that cannot be sub-classed. Final classes are declared using the keyword 'final'.
Final methods are methods that cannot be overridden by sub-classes. Final methods are declared using the keyword 'final'...
*** See complete answer and code snippet in the Java Interview Guide.
What are Access and Non-Access modifiers that can be added to a class or class-member declaration?
FAQ* Similar Java Interview Question Recently Asked @ ADP, Argonne National Laboratory, BAE Systems, Guidewire, Impetus Technologies, Polaris, Virtusa,
Access modifiers control how a class or its members can be accessed. Non-access modifiers control other aspests of a class and its members.
For your interview you have to know the following combination of modifiers.
1. Class access modifiers
Class non-access modifiers
3. Class-member access modifiers
4. Class-member non-access modifiers.
The next four questions addresses these combinations.
What are the access modifiers that can be added to a class?
FAQFollowing access modifiers can be added to a class declaration.
public - A class declared with public access modifier is accessible to all classes.
*** See complete answer and code snippet in the Java Interview Guide.
What are the Non-access modifiers that can be added to a class declaration?
FAQFollowing non-access modifiers can be added to a class declaration.
final - A class declared as final cannot be sub-classed...
*** See complete answer and code snippet in the Java Interview Guide.
Java Interview Guide has over 250 REAL questions from REAL interviews. Get the guide for $15.00 only.
 
BUY EBOOK 
What are the access modifiers that can be added to members of a Class?
FAQFollowing are the access modifiers that can be added to members of a class,
public - A class member declared with public access modifier is visible and accessible to all classes.
*** See complete answer and code snippet in the Java Interview Guide.
What are the non-access modifiers that can be added to members of a Class?
FAQKey ConceptFollowing are the non-access modifiers that can be added to methods of a class.
final - A final method cannot be overridden by a sub-class...
*** See complete answer and code snippet in the Java Interview Guide.